Want to level up your web development skills? Node.js is your perfect companion! This powerful tool lets developers use JavaScript on both frontend and backend, making web development smoother and faster. Thanks to its rich features and huge collection of npm packages, Node.js has become a must-have for modern web developers. Whether you’re just starting out or already coding like a pro, learning Node.js will help you build better, faster websites that can handle lots of users with ease.
Understanding Advanced Modules in Node.js
Think of Node.js modules as LEGO blocks for your code! These handy pieces of code can be easily reused across your projects with a simple “require” command. From basic functions to powerful libraries, modules help keep your code clean and organized. Just like organizing your closet makes finding clothes easier, modules make your code more manageable and help it grow smoothly as your project gets bigger.
Importance of Modules in Node.js
Modules are a core component in Node.js. They help in breaking down complex code into simpler, manageable, reusable pieces, thereby enhancing code maintainability and reducing the chance of bugs. Modules encapsulate functionality into separate units of code, which can be independently developed and easily updated without impacting other parts of the program. This model promotes cleaner, more readable code, and allows developers to reuse functionality across different parts of an application or across different projects entirely.
Creating and Using Custom Modules
Creating custom modules in Node.js allows developers to write more personalized and optimized code for their specific needs. Here are some steps to create a custom module:
- Create a new JavaScript file, e.g., myModule.js.
- Encapsulate the desired functionality within the module.exports object. For example:
module.exports = {
greet: function(name) {
console.log(\`Hello, ${name}\`);
}
};
- Save the module, then import and use it in other parts of your Node.js application using the require syntax:
var myModule = require('./myModule');
myModule.greet('Alice');
By creating custom modules, developers gain control over their functionality and can easily integrate it wherever it’s needed in their application. Plus, sharing and using modules across different projects becomes a breeze, promoting code reuse and reducing the time needed for development.
Mastering the Event Loop
The event loop is a fundamental aspect of Node.js, allowing it to perform non-blocking I/O operations, despite JavaScript being single-threaded. At its core, the event loop enables Node.js to execute multiple operations in the background—such as reading from the network or accessing the file system—while continuing to run the main program code without interruption. This architecture maximizes efficiency and throughput in server-side applications by ensuring that slow operations don’t stall the main thread.
Implementing Asynchronous Operations with Event Loop
To leverage the power of the event loop, Node.js uses asynchronous functions that handle operations like reading files, API calls, or querying a database. These functions take callbacks, promises, or use async/await syntax to defer actions until the operation completes. Here’s how it typically works:
- A request is made to read a file.
- Node.js sends the file reading task to the event loop.
- While the file is being read (an I/O operation), Node.js can handle other tasks.
- Once the file is read, the callback function is executed with the data from the file.
This non-blocking nature ensures that Node.js applications can handle a high volume of operations, making them highly scalable.
Avoiding Blocking Code with Event Loop
To maintain the efficiency of the event loop, it’s crucial to avoid blocking code, or synchronous operations, that can tie up the single thread. Examples of blocking code include loops that wait for operations to complete before proceeding, or synchronous I/O operations. Instead, developers should use non-blocking versions and understand the importance of returning control to the event loop regularly. This practice notates robust, responsive applications that can serve many users or tasks concurrently.
Advanced Error Handling in Node.js
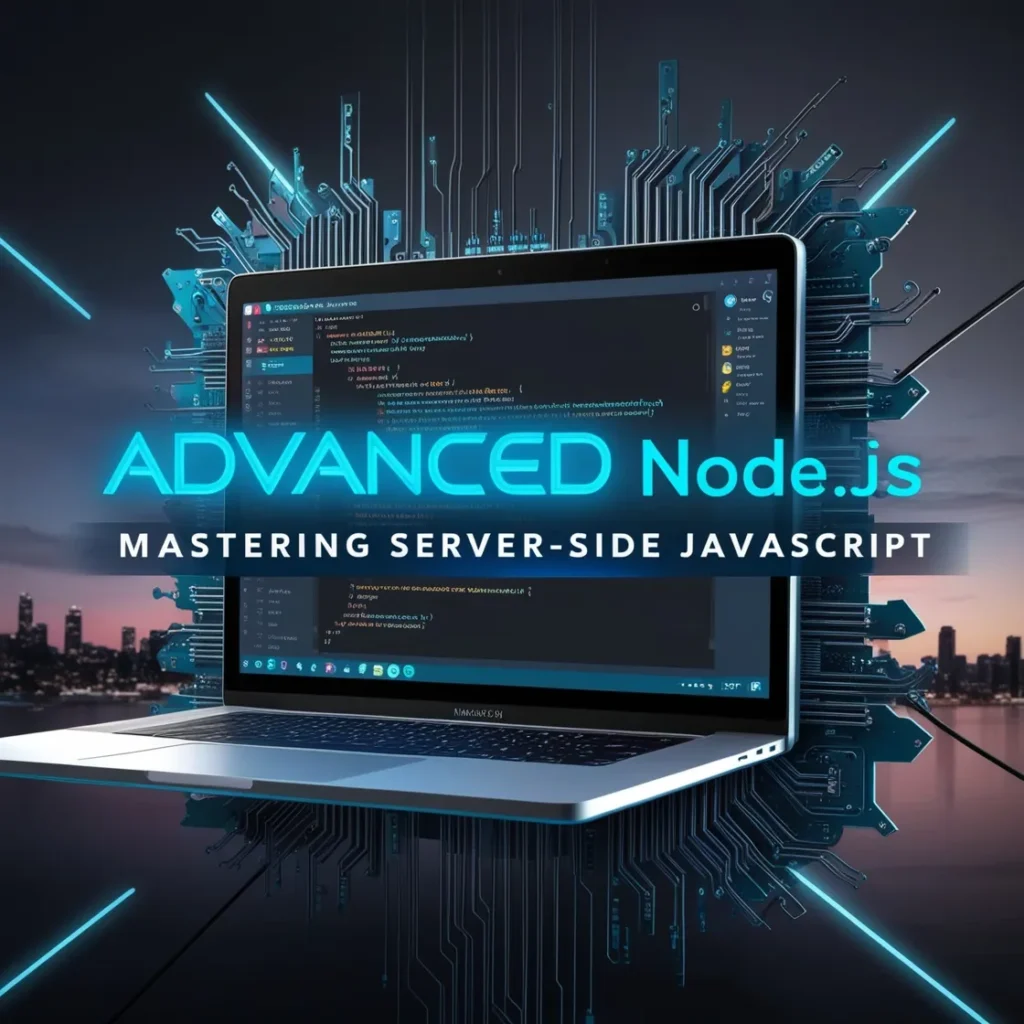
Node.js applications can encounter a variety of common errors, such as network errors, file system errors, or errors due to incorrect application logic. Understanding these errors is the first step towards robust error handling. Network errors might include connection timeouts or disrupted transfers. File system errors generally arise from problems in reading or writing files. Logical errors could be due to incorrect conditions or data processing failures.
Using Try-Catch and Error Objects for Error Handling
Effective error handling in Node.js often involves the use of try-catch statements and error objects. These tools help manage exceptions and maintain application stability by catching runtime errors before they cause the application to crash.
- Try-Catch: This structure allows you to test a block of code for errors while it’s being executed.
- Error Objects: When an error occurs, Node.js throws an Error object that can provide valuable information, including the error name, message, and stack trace.
Developers can also customize error responses by defining error-handling middleware especially in Express applications, thus improving the user experience and debugging process. By mastering these error handling techniques, Node.js developers can ensure smoother, more reliable application performance, even when facing unexpected issues.
Security Best Practices in Node.js
Node.js, like any other powerful tool, comes with its set of security challenges that must be acknowledged and addressed to protect applications from potential threats. Common security risks include SQL injection, Cross-Site Scripting (XSS), and Cross-Site Request Forgery (CSRF). These vulnerabilities primarily arise due to improper input validation and malicious code injections. Additionally, since Node.js applications are often used for handling real-time data over web sockets, they can be particularly susceptible to Denial-of-Service (DoS) attacks. Understanding these risks is the first step toward mitigating them and improving the security posture of Node.js applications.
Implementing Security Measures in Node.js Applications
Once the potential security threats have been identified, taking proactive steps to implement robust security measures is essential. Here are some effective strategies to enhance the security of Node.js applications:
- Validation and Sanitization: Ensure all input is validated and sanitized to prevent SQL injections and XSS attacks.
- Securing HTTP headers: Use modules like \`helmet\` to set HTTP headers appropriately and avoid exposing sensitive application information.
- Managing Dependencies: Regularly update and audit Node modules to protect against vulnerabilities found in outdated packages.
- Implementing Authentication and Authorization Practices: Tools like OAuth and JSON Web Tokens (JWT) can help manage user authentication and maintain secure API access.
- Regular Security Audits: Regularly conduct code reviews and security audits to detect and remedy security weaknesses early.
By combining a deep understanding of the theoretical aspects through practice lectures and video tutorials with the execution of these robust security practices, developers can dramatically enhance the security and efficiency of their Node.js applications.
Performance Optimization Techniques
Optimizing performance starts by pinpointing where the bottlenecks occur within your Node.js applications. This can be achieved through profiling tools and monitoring solutions that help trace and analyze the operations of your application. Common tools for this purpose include Node.js built-in profiler, Google Chrome’s DevTools, and third-party services like New Relic or Dynatrace. The data collected will often highlight issues such as slow I/O operations, heavy computation tasks, or inefficient database queries. It’s important to interpret these details accurately to prioritize the most impactful optimizations.
Implementing Caching for Performance Improvement
Caching is a crucial strategy to enhance the performance of Node.js applications by storing previously fetched data. This technique significantly reduces the need to perform costly operations repeatedly. Implementing caching can be done at various levels:
- In-memory caches such as Redis can store session information or frequently accessed items.
- Database query results caching to avoid redundant queries for common requests.
- Reverse proxy caches like Varnish can be used to cache entire web pages or API responses at the network edge.
Appropriate use of caching not only improves response times but also helps in reducing the load on the server, making the application faster and more responsive.
Utilizing Load Balancing for Scalability
For Node.js applications that experience high traffic, load balancing is essential to distribute the incoming network traffic across multiple servers or instances. This prevents any single server from becoming a bottleneck, thereby enhancing the application’s scalability and reliability. Techniques like round-robin DNS, or using dedicated load balancers such as NGINX or HAProxy, can efficiently manage traffic. Furthermore, integrating with cloud providers like AWS or Azure, which offer built-in load balancing solutions, simplifies scalability while providing robust uptime and performance.
Conclusion
Mastering advanced Node.js topics such as performance optimization not only enhances your applications but also ensures they can scale and perform well under increased loads. By focusing on identifying bottlenecks, implementing strategic caching, and utilizing effective load balancing, developers can maximize the efficiency and reliability of their server-side JavaScript applications. Embracing these sophisticated techniques will equip you to tackle more complex projects and meet modern demands in the dynamic world of web development.
Pingback: Boost Your Node.js Performance with Express Middleware
Pingback: Navigating the Latest Node.js Syllabus Updates: What’s New?
Pingback: Understanding Event-Emitter in Node.js A Comprehensive Guide
Pingback: JavaScript Syllabus for Beginners & Advanced