The landscape of JavaScript development has undergone a remarkable transformation over the past decade. What started as a simple browser scripting language has evolved into a powerful tool for building complex server-side applications. At the heart of this evolution lies a fascinating story of two platforms: Node.js and Deno.
When Ryan Dahl introduced Node.js in 2009, it revolutionized how developers thought about JavaScript. For the first time, JavaScript could break free from the constraints of the browser and run directly on servers. This breakthrough moment marked the beginning of a new era in web development, where JavaScript could handle both front-end and back-end responsibilities seamlessly.
Fast forward to 2020, and the same creator introduced Deno, positioning it as a modern runtime that addresses many of Node.js’s historical limitations. This arrival sparked intense discussion in the development community about the future of server-side JavaScript. Now in 2024, both platforms have carved out their own niches, each offering unique advantages for different types of projects.
The Evolution of Node.js
Node.js emerged from a simple yet powerful idea: what if developers could use the same language they used in the browser to write server-side code? This concept resonated deeply with the development community. Built on Chrome’s V8 engine, Node.js introduced an event-driven, non-blocking I/O model that proved perfect for building scalable network applications.
The platform’s success story isn’t just about its technical capabilities. The introduction of npm (Node Package Manager) created an ecosystem that would grow to become the world’s largest software registry. This vast collection of reusable code modules transformed how developers build applications, making it possible to construct complex systems by combining existing packages rather than writing everything from scratch.
Consider how Node.js handles asynchronous operations. Traditional server-side languages often block execution while waiting for operations like file reading or network requests to complete. Node.js takes a different approach, as demonstrated in this real-world scenario:

Key Features
- Event-Driven Architecture Node.js is like a great multitasker. Instead of waiting for one task to finish before starting another, it can handle multiple tasks simultaneously. Imagine a waiter taking orders from multiple tables without waiting for one table’s food to be prepared before moving to the next.
- NPM (Node Package Manager) Think of NPM as the world’s largest software library. It’s like having access to millions of pre-built Lego pieces that you can use in your projects. Need to handle dates? There’s Moment.js. Need to build a web server? Express.js has got you covered.
- Rich Ecosystem The Node.js ecosystem is like a thriving city with everything you might need. Here’s a practical example of using popular packages:
const fs = require('fs');
const server = require('http').createServer();
server.on('request', (req, res) => {
fs.readFile('large-file.txt', (err, data) => {
if (err) throw err;
// Process data while handling other requests
res.end(data);
});
// This code runs immediately, without waiting for file read
console.log('Handling new request');
});
server.listen(8000);
This non-blocking approach has made Node.js particularly well-suited for applications requiring real-time interactions, such as chat applications, streaming services, and collaborative tools.
Understanding Deno’s Modern Approach
When Ryan Dahl introduced Deno, he wasn’t just creating another JavaScript runtime; he was addressing fundamental issues he’d observed during his years working with Node.js. Deno represents a fresh start, built with modern development practices in mind and a strong emphasis on security.
The security-first approach of Deno fundamentally changes how developers interact with system resources. Unlike Node.js, where scripts have unrestricted access to the file system and network by default, Deno requires explicit permissions. This approach might seem restrictive at first, but it prevents many common security vulnerabilities before they can occur.
Key Features
- Built-in TypeScript Support While Node.js needs extra setup for TypeScript, Deno speaks it natively. It’s like having a car that comes with all the premium features built-in, no additional installation required.
- Security First Deno is like a cautious security guard. By default, it doesn’t trust any code with access to your system. Want to access files or the network? You need explicit permission:
Here’s how Deno handles secure file operations:
// This won't work without explicit permissions
try {
const data = await Deno.readFile("sensitive.txt");
console.log(new TextDecoder().decode(data));
} catch (error) {
console.error("Permission denied: Run with --allow-read flag");
}
Deno’s built-in TypeScript support represents another significant advancement. While Node.js requires additional configuration and tools to work with TypeScript, Deno treats it as a first-class citizen. This integration simplifies the development process and provides better type safety out of the box.
The Module System Revolution
One of the most striking differences between Node.js and Deno lies in their approach to module management. Node.js relies on the npm ecosystem, with its package.json files and node_modules directories. This system, while powerful, has been criticized for its complexity and potential security issues.
Deno takes a radically different approach, using URLs for module imports. This method might seem unusual to Node.js developers, but it offers several advantages. Module versioning becomes explicit, and dependencies are loaded on demand. Here’s how it works in practice:
import { serve } from "https://deno.land/std@0.177.0/http/server.ts";
import { graphql } from "https://deno.land/x/gql@1.1.0/mod.ts";
const server = serve({ port: 8000 });
console.log("Server running on http://localhost:8000/");
for await (const req of server) {
// Handle requests
}
Performance and Production Readiness
In the real world, performance matters, and both platforms have their strengths. Node.js benefits from years of optimization and production hardening. Its performance characteristics are well-understood, and there are established patterns for solving common problems.
Deno, being built with modern requirements in mind, often shows better performance in specific scenarios, particularly when dealing with TypeScript and modern JavaScript features. However, the real performance consideration often comes down to the ecosystem and tools available for solving specific problems.
For instance, here’s how both platforms handle a common web server scenario:
const express = require('express');
const app = express();
app.get('/api/users', async (req, res) => {
const users = await database.getUsers();
res.json(users);
});
app.listen(3000);
Deno with Oak (a similar web framework):
import { Application } from "https://deno.land/x/oak/mod.ts";
const app = new Application();
app.use(async (ctx) => {
const users = await database.getUsers();
ctx.response.body = users;
});
await app.listen({ port: 3000 });
Package Management
Node.js Package Management
// package.json
{
"dependencies": {
"express": "^4.17.1",
"lodash": "^4.17.21"
}
}
// Installing
// npm install
// Usage
const express = require('express');
Deno Package Management
// Direct URL imports
import { serve } from "https://deno.land/std/http/server.ts";
import _ from "https://deno.land/x/lodash@4.17.21/lodash.js";
Pros and Cons
Node.js Advantages
- Mature Ecosystem
- Millions of packages available
- Extensive documentation
- Large community support
- Battle-tested in production
- Wide Adoption
- Used by major companies
- Easy to find developers
- Abundant learning resources
- Tooling
- Rich development tools
- Extensive IDE support
- Many deployment options
Node.js Disadvantages
- Security Concerns
- No built-in security features
- Dependency vulnerabilities
- Manual security implementation needed
- Legacy Baggage
- Historical design decisions
- Callback hell in older code
- Complex module system
Deno Advantages
- Modern Features
- Built-in TypeScript support
- Modern JavaScript features
- Better standard library
- Security
- Permissions system
- Secure by default
- Better module security
- Built-in Tools
- Testing
- Documentation
- Code formatting
Deno Disadvantages
- Smaller Ecosystem
- Fewer packages available
- Less community support
- Fewer production examples
- Learning Curve
- Different from Node.js
- New concepts to learn
- Fewer tutorials available
Which Should You Choose?
Choose Node.js When:
- Building Enterprise Applications
- You need proven reliability
- Large team collaboration is required
- Extensive third-party integration is needed
Example use case:
// Complex enterprise API with multiple integrations
const express = require('express');
const mongoose = require('mongoose');
const redis = require('redis');
const app = express();
// Easy integration with various services
mongoose.connect('mongodb://localhost/myapp');
const redisClient = redis.createClient();
app.get('/api/data', async (req, res) => {
// Complex business logic with multiple dependencies
});
- Need Maximum Package Availability
- Specific package requirements
- Complex development requirements
- Legacy system compatibility
- Team Expertise
- Team familiar with Node.js
- Easy to find developers
- Extensive documentation available
Choose Deno When:
- Security is Priority
- Building security-sensitive applications
- Need fine-grained permissions
- Want secure defaults
Example use case:
// Secure API with TypeScript
import { Application } from "https://deno.land/x/oak/mod.ts";
const app = new Application();
app.use(async (ctx) => {
// TypeScript support out of the box
const data: Array<string> = await secureOperation();
ctx.response.body = data;
});
await app.listen({ port: 8000 });
- TypeScript-First Development
- Heavy TypeScript usage
- Modern JavaScript features
- Clean codebase priority
- New Projects
- No legacy constraints
- Modern architecture
- Small to medium scale
Making the Choice: Real-world Considerations
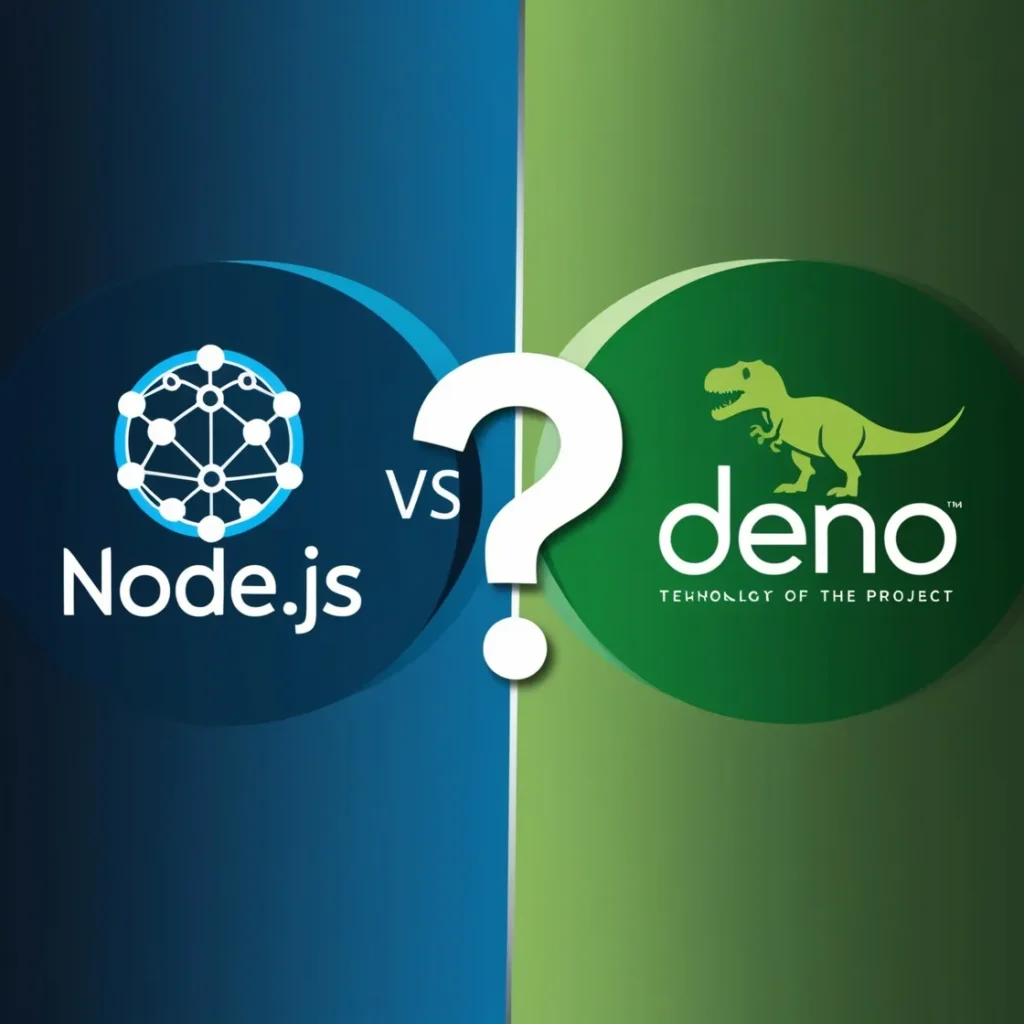
Choosing between Node.js and Deno isn’t just about technical features; it’s about your specific needs and constraints. Node.js remains an excellent choice for enterprise applications, particularly when you need access to a vast ecosystem of packages and tools. Its mature ecosystem means you’re likely to find solutions for almost any problem you encounter.
Deno shines in scenarios where security is paramount, or when you’re starting a new project that can benefit from modern JavaScript features and TypeScript integration. Its security-first approach and built-in development tools make it particularly attractive for teams that value these features.
Conclusion
The choice between Node.js and Deno reflects a broader evolution in how we think about server-side JavaScript development. Node.js continues to excel in enterprise environments and complex applications where its vast ecosystem provides immediate solutions to common problems. Its maturity and widespread adoption make it a safe choice for teams that need proven reliability and extensive third-party support.
Deno represents the future of server-side JavaScript, with its emphasis on security, modern language features, and improved developer experience. For new projects that can take advantage of these features, Deno offers a compelling platform that addresses many of the historical pain points of Node.js development.
Ultimately, both platforms have their place in the modern development landscape. Instead of viewing them as competitors, consider them as complementary tools that serve different needs and use cases. As the JavaScript ecosystem continues to evolve, having knowledge of both platforms will become increasingly valuable for developers looking to build robust, secure, and maintainable applications.
Whether you choose Node.js or Deno, the key is to understand your project’s specific requirements and constraints. Consider factors like team expertise, security requirements, deployment environment, and the need for specific packages or tools. With this understanding, you can make an informed decision that best serves your project’s needs.